Using the Phalcon PHP Framework with MySQL
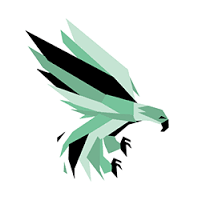
PHP—a recursive acronym for PHP Hypertext Preprocessor—is an open source, object-oriented, interpreted, server-side scripting language for developing dynamic websites. PHP is the number-one server-side scripting language, and it’s used in design and development of almost 75 percent of websites.
However, one of the features that had been lacking in PHP is built-in support for the model-view-controller (MVC) design pattern. Without a pre-installed PHP extension that supports MVC, that pattern has to be implemented externally.
Some PHP frameworks are now available that support the MVC design pattern, most notable among them being the open source Phalcon web framework. Phalcon provides the lowest level of overhead incurred due to memory and CPU for MVC applications. For data storage and access, Phalcon provides object-relational mapping (ORM) features. Open source databases MySQL, PostgreSQL, and SQLite are supported out of the box.
Let’s look at how to use Phalcon’s MVC and ORM features with the popular MySQL database.
First, install the Phalcon extension in php.ini:
extension=php_phalcon.dll
Also activate the MySQLi extension, which is used to connect to the MySQL database.
Next, create a MySQL database table using the MySQLi extension. The PHP script used to create a database table would be similar to this script listed on GitHub.
Phalcon supports PHP’s auto-loading mechanism for classes. Create a bootstrap file to configure the Phalcon framework, and import the requisite classes and namespaces. The Phalcon\Loader class lets you autoload project classes using PHP's autoloading mechanism.
Define some absolute path constants for locating resources. Create an instance of the autoloader and register directories in which model, view, and controller files are located, and create an instance of the dependency injection container. You’ll also need to create a database service. The bootstrap file is listed on GitHub as /public/index.php.
URLs can be rather complex when developing a web application, and the Apache HTTP server provides a module to be able to configure rewrite rules for modifying URLs. To configure rewrite rules, load the rewrite module using a .htaccess file.
Create a Phalcon MVC controller that specifies an index controller action, and invoke the controller action to output a “Hello!” message:
<?php
use Phalcon\Mvc\Controller;
class IndexController extends Controller
{
public function indexAction()
{
echo '<h1>Hello!</h1>';
}
}
?>
Next, use Phalcon MVC views (.phtml) to create and find a catalog entry in the MySQL database. Add a Phalcon view at index.phtml to add a catalog entry. The user interface consists of fields for specifying the different attributes of a catalog entry.
Then, add a Phalcon MVC model class called Catalog by extending the Phalcon\Mvc\Model class.
You’ll also need to add a controller action in the index controller to save a catalog entry in the database. Use an instance of the Catalog to create a catalog entry.
Next, invoke the controller action to create and save a catalog entry:
Query the database table using model’s find() method and display the result in a browser:
If you use PHP, Phalcon is great for enabling you to develop MVC applications. It’s a high-performance framework with low memory usage, which makes it an efficient choice, particularly when used with the MySQL database.